Full Stack Data Science
Full-stack data science covers the entire spectrum of data handling, from gathering and manipulating data to backend processing, model deployment, and the implementation of complete machine learning solutions including natural language processing (NLP), computer vision (CV), and traditional machine learning algorithms. This approach integrates the entire pipeline of data science workflows into a cohesive skill set, allowing professionals to manage and execute projects from start to finish.
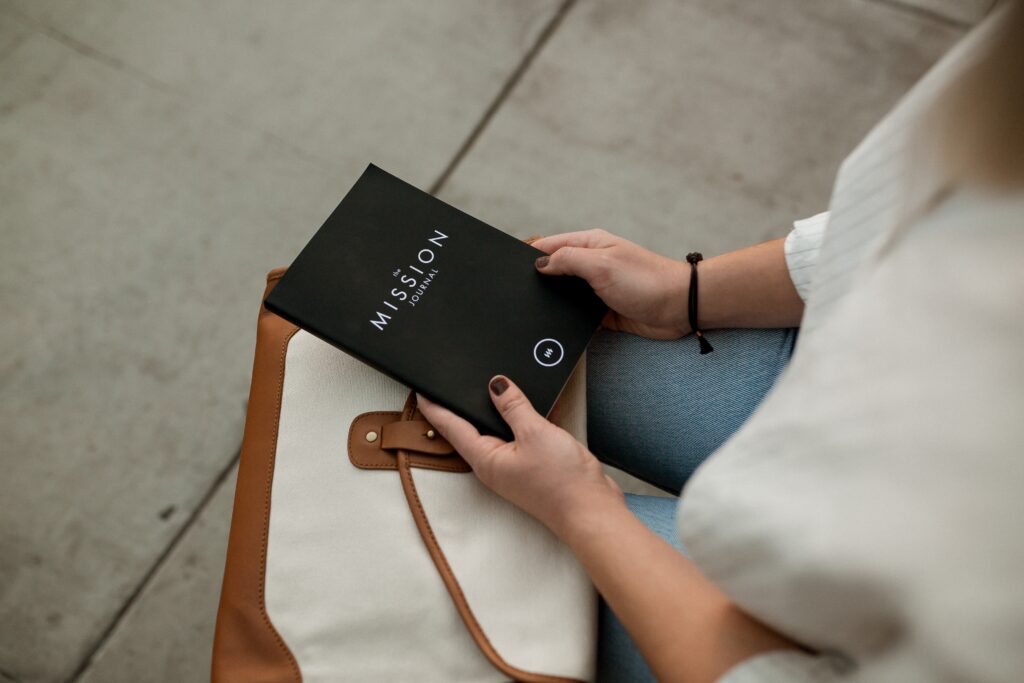
Skills You Will Gain
Machine Learning
Deep Learning
SQL
DJango
NLP
Wordcloud
ANN
Python
LSTM
Database
Docker
Flask
Redis
Kafka
Numpy
Image Processing
CNN
This course includes
- 1 : 1 Session
- 100% Placement Assistance
- 35 Weeks + Projects
- Real TIme Project Training
Syllabus Overview
Basic of Python
- Introduction to Python
- What is Python and why use it?
- Setting up the Python environment
- Basic syntax and execution flow
- Writing your first Python script
- Variables and Data Types
- Understanding variables and basic data types (integers, floats, strings)
- Type casting and data type conversion
- Control Flow
- Making decisions with if, elif, and else
- Looping with for and while
- Controlling loop flow with break and continue
- Data Structures (Part 1)
- Lists: Creation, indexing, and list operations
- Tuples: Immutability and tuple operations
- Data Structures (Part 2)
- Sets: Usage and set operations
- Dictionaries: Key-value pairs, accessing, and manipulating data
- Functions
- Defining functions and returning values
- Function arguments and variable scope
- Anonymous functions: Using lambda
- File Handling
- Reading from and writing to files
- Handling different file types (text, CSV, etc.)
- Error Handling and Exceptions
- Try and except blocks
- Raising exceptions
- Using finally for cleanup actions
- Object-Oriented Programming (OOP)
- Classes and objects: The fundamentals
- Encapsulation: Private and protected members
- Inheritance: Deriving classes
- Polymorphism: Method overriding
- Advanced Data Structures
- List comprehensions for concise code
- Exploring the collections module: Counter, defaultdict, OrderedDict
- Decorators and Context Managers
- Creating and applying decorators
- Managing resources with context managers and the with statement
- Concurrency
- Introduction to concurrency with threading
- Understanding the Global Interpreter Lock (GIL)
- Basics of asynchronous programming with asyncio
- Introduction to Excel
- Overview of Excel’s interface and features
- Basic spreadsheet operations: entering data, formatting cells, sorting and filtering
- Introduction to formulas and cell references
- Summarizing data with SUM, AVERAGE, MIN, MAX, COUNT
- Working with Data
- Data types and best practices for data entry
- Using ranges, tables, and data validation
- Understanding date and time functions
- Conditional functions like IF, COUNTIF, SUMIF
- Mastering Excel Functions
- Exploring logical functions: AND, OR, NOT
- Mastering lookup functions: VLOOKUP, HLOOKUP, INDEX, MATCH
- Nesting functions for complex calculations
- Text functions to manipulate strings
- Data Visualization
- Creating and customizing charts
- Using conditional formatting to highlight data
- Introduction to PivotTables for summarizing data
- PivotCharts and slicers for interactive reports
- Introduction to SQL and Database Concepts
- Overview of relational databases
- Basic SQL syntax and setup
- SELECT and FROM clauses to retrieve data
- Sorting and filtering data with ORDER BY and WHERE
- Working with SQL Joins and Aggregations
- Understanding different types of joins: INNER, LEFT, RIGHT, and FULL
- Using aggregate functions like COUNT, SUM, AVG, MIN, and MAX
- Grouping data with GROUP BY
- Filtering grouped data using HAVING
- Advanced SQL Operations
- Subqueries: using subqueries in SELECT, FROM, and WHERE clauses
- Common Table Expressions (CTEs) and WITH clause
- Advanced data manipulation with INSERT, UPDATE, DELETE, and MERGE
- Mastering SQL Functions and Complex Queries
- String functions, date functions, and number functions
- Conditional logic in SQL with CASE statements
- Advanced use of data types and casting
- Exploring SQL Window Functions
- Introduction to window functions
- Using OVER() with PARTITION BY, ORDER BY
- Functions like ROW_NUMBER(), RANK(), DENSE_RANK(), LEAD(), LAG()
- SQL Performance Tuning
- Understanding indexes, including when and how to use them
- Query optimization techniques
- Using EXPLAIN plans to analyze query performance
- Transaction Management and Security
- Understanding transactions, ACID properties
- Implementing transaction control with COMMIT, ROLLBACK
- Basics of database security: permissions, roles
- Integrating SQL with Other Technologies
- Linking SQL databases with programming languages like Python
- Using SQL data in Excel via ODBC, direct queries
- Introduction to using APIs with SQL databases for web integration
- Advanced Data Analytics Tools in SQL
- Using analytical functions for deeper insights
- Exploring materialized views for performance
- Dynamic SQL for flexible query generation
- Capstone Project
- Designing and implementing a database schema for a real-world application
- Comprehensive data analysis using advanced SQL techniques
- Integrating SQL knowledge with tools like Python and Excel to provide business solutions
- Presenting findings and insights effectively
- Understanding NoSQL
- Overview of NoSQL
- Definition and evolution of NoSQL databases.
- Differences between NoSQL and traditional relational database systems (RDBMS).
- Types of NoSQL Databases
- Key-value stores, document stores, column stores, and graph databases.
- Use cases and examples of each type.
- Overview of NoSQL
- NoSQL Concepts and Data Models
- NoSQL Data Modeling
- Understanding NoSQL data modeling techniques.
- Comparing schema-on-read vs. schema-on-write.
- Advantages of NoSQL
- Scalability, flexibility, and performance considerations.
- When to choose NoSQL over a traditional SQL database.
- NoSQL Data Modeling
- Getting Started with MongoDB
- Installing MongoDB
- Setting up MongoDB on different operating systems.
- Understanding MongoDB’s architecture: databases, collections, and documents.
- Basic Operations in MongoDB
- CRUD (Create, Read, Update, Delete) operations.
- Using the MongoDB Shell and basic commands.
- Installing MongoDB
- Working with Data in MongoDB
- Data Manipulation
- Inserting, updating, and deleting documents.
- Querying data: filtering, sorting, and limiting results.
- Indexing and Aggregation
- Introduction to indexing for performance improvement.
- Basic aggregation operations: $sum, $avg, $min, $max, and $group.
- Data Manipulation
- Introduction to Statistics
- Overview of Statistics in Data Science
- Role of statistics in data analysis and machine learning.
- Differentiation between descriptive and inferential statistics.
- Basic Statistical Measures
- Measures of central tendency (mean, median, mode).
- Measures of dispersion (variance, standard deviation, range, interquartile range).
- Overview of Statistics in Data Science
- Probability Fundamentals
- Probability Concepts
- Basic probability rules, conditional probability, and Bayes’ theorem.
- Probability Distributions
- Introduction to normal, binomial, Poisson, and uniform distributions.
- Probability Concepts
- Hypothesis Testing
- Concepts of Hypothesis Testing
- Null hypothesis, alternative hypothesis, type I and type II errors.
- Key Tests
- t-tests, chi-square tests, ANOVA for comparing group means.
- Concepts of Hypothesis Testing
- Regression Analysis
- Linear Regression
- Simple and multiple linear regression analysis.
- Assumptions of linear regression, interpretation of regression coefficients.
- Logistic Regression
- Understanding logistic regression for binary outcomes.
- Linear Regression
- Multivariate Statistics
- Advanced Regression Techniques
- Polynomial regression, interaction effects in regression models.
- Principal Component Analysis (PCA)
- Reducing dimensionality, interpretation of principal components.
- Advanced Regression Techniques
- Time Series Analysis
- Fundamentals of Time Series Analysis
- Components of time series data, stationarity, seasonality.
- Time Series Forecasting Models
- ARIMA models, seasonal decompositions.
- Fundamentals of Time Series Analysis
- Bayesian Statistics
- Introduction to Bayesian Statistics
- Bayes’ Theorem revisited, prior and posterior distributions.
- Applied Bayesian Analysis
- Using Bayesian methods in data analysis and prediction.
- Introduction to Bayesian Statistics
- Non-Parametric Methods
- Overview of Non-Parametric Statistics
- When to use non-parametric methods, advantages over parametric tests.
- Key Non-Parametric Tests
- Mann-Whitney U test, Kruskal-Wallis test, Spearman’s rank correlation.
- Overview of Non-Parametric Statistics
- Introduction to Exploratory Data Analysis
- Overview of EDA
- The importance and objectives of EDA.
- Key steps in the EDA process.
- Overview of EDA
- Data Handling with Pandas
- Getting Started with Pandas
- Introduction to Pandas DataFrames and Series.
- Reading and writing data with Pandas (CSV, Excel, SQL databases).
- Data Cleaning Techniques
- Handling missing values.
- Data type conversions.
- Renaming and replacing data.
- Data Manipulation
- Filtering, sorting, and grouping data.
- Merging and concatenating datasets.
- Advanced operations with groupby and aggregation.
- Getting Started with Pandas
- Numerical Analysis with NumPy
- Introduction to NumPy
- Creating and manipulating arrays.
- Array indexing and slicing.
- Statistical Analysis with NumPy
- Basic statistics: mean, median, mode, standard deviation.
- Correlations and covariance.
- Generating random data and sampling.
- Introduction to NumPy
- Visualization Techniques
- Using Matplotlib
- Basics of creating plots, histograms, scatter plots.
- Customizing plots: colors, labels, legends.
- Advanced Visualization with Seaborn
- Statistical plots in Seaborn: box plots, violin plots, pair plots.
- Heatmaps and clustermaps.
- Facet grids for multivariate analysis.
- Using Matplotlib
- Introduction to Machine Learning
- Overview of Machine Learning
- Definitions and Significance: Students will explore the fundamental concepts and various definitions of machine learning, understanding its crucial role in leveraging big data in numerous industries such as finance, healthcare, and more.
- Types of Machine Learning: The course will differentiate between the three main types of machine learning: supervised learning (where the model is trained on labeled data), unsupervised learning (where the model finds patterns in unlabeled data), and reinforcement learning (where an agent learns to behave in an environment by performing actions and receiving rewards).
- Overview of Machine Learning
- Supervised Learning Algorithms
- Regression Algorithms
- Linear Regression: Focuses on predicting a continuous variable using a linear relationship formed from the input variables.
- Polynomial Regression: Extends linear regression to model non-linear relationships between the independent and dependent variables.
- Decision Tree Regression: Uses decision trees to model the regression, helpful in capturing non-linear patterns with a tree structure.
- Classification Algorithms
- Logistic Regression: Used for binary classification tasks; extends to multiclass classification under certain methods like one-vs-rest (OvR).
- K-Nearest Neighbors (KNN): A non-parametric method used for classification and regression; in classification, the output is a class membership.
- Support Vector Machines (SVM): Effective in high-dimensional spaces and ideal for complex datasets with clear margin of separation.
- Decision Trees and Random Forest: Decision Trees are a non-linear predictive model, and Random Forest is an ensemble method of Decision Trees.
- Naive Bayes: Based on Bayes’ Theorem, it assumes independence between predictors and is particularly suited for large datasets.
- Regression Algorithms
- Ensemble Methods and Handling Imbalanced Data
- Ensemble Techniques
- Detailed techniques such as Bagging (Bootstrap Aggregating), Boosting, AdaBoost (an adaptive boosting method), and Gradient Boosting will be covered, emphasizing how they reduce variance and bias, and improve predictions.
- Strategies for Imbalanced Data
- Techniques such as Oversampling, Undersampling, and Synthetic Minority Over-sampling Technique (SMOTE) are discussed to handle imbalanced datasets effectively, ensuring that the minority class in a dataset is well-represented and not overlooked.
- Ensemble Techniques
- Unsupervised Learning Algorithms
- Clustering Techniques
- K-Means Clustering: A method of vector quantization, originally from signal processing, that aims to partition n observations into k clusters.
- Hierarchical Clustering: Builds a tree of clusters and is particularly useful for hierarchical data, such as taxonomies.
- DBSCAN: Density-Based Spatial Clustering of Applications with Noise finds core samples of high density and expands clusters from them.
- Association Rule Learning
- Apriori and Eclat algorithms: Techniques for mining frequent item sets and learning association rules. Commonly used in market basket analysis.
- Clustering Techniques
- Model Evaluation and Hyperparameter Tuning
- Evaluation Metrics
- Comprehensive exploration of metrics such as Accuracy, Precision, Recall, F1 Score, and ROC-AUC for classification; and MSE, RMSE, and MAE for regression.
- Hyperparameter Tuning
- Techniques such as Grid Search, Random Search, and Bayesian Optimization with tools like Optuna are explained. These methods help in finding the most optimal parameters for machine learning models to improve performance.
- Evaluation Metrics
- Introduction to Python and NumPy
- Python Basics
- Review of Python syntax, data structures, and essential programming concepts.
- NumPy Mastery
- Deep dive into NumPy for scientific computing: array manipulations, broadcasting, and vectorized operations.
- Python Basics
- Foundations of Tensor Manipulation
- TensorFlow and PyTorch Basics
- Introduction to these frameworks for deep learning, focusing on their tensor operations.
- Fundamental Tensor Operations
- Creating tensors, understanding shapes, indexing, and slicing. Basic mathematical operations and their applications in data processing.
- TensorFlow and PyTorch Basics
- Introduction to Neural Networks
- Neural Network Architecture
- Detailed explanation of neurons, layers, weights, biases, and activation functions.
- Building Simple Neural Networks
- Step-by-step guide to building and training a basic neural network for a binary classification problem.
- Neural Network Architecture
- Introduction to Convolutional Neural Networks
- Convolutional Layers Explained
- Understanding convolutions, the role of filters, and feature map generation.
- Pooling and Normalization
- Techniques like max pooling and batch normalization to improve model training dynamics.
- Convolutional Layers Explained
- Implementing and Training CNNs
- CNN Architectures
- Construction of CNNs using PyTorch or TensorFlow for image classification tasks.
- Training CNNs
- Detailed process of training, including setting up loss functions, optimizers, and learning rate schedules.
- CNN Architectures
- Advanced CNN Architectures
- Deep Dive into VGG, ResNet, and Inception
- Exploration of these architectures’ designs, innovations, and impact on the field of deep learning.
- Hands-On Implementation
- Practical implementation and comparison of these models using standard datasets like CIFAR-10 or ImageNet.
- Deep Dive into VGG, ResNet, and Inception
- Object Detection and Recognition
- Object Detection Frameworks
- Study of R-CNN, Fast R-CNN, Faster R-CNN, YOLO, and SSD architectures.
- Practical Application
- Implementing an object detection model to recognize and localize multiple objects in an image.
- Object Detection Frameworks
- Generative Adversarial Networks (GANs) and Applications
- Understanding GANs
- Concepts behind GANs, including the roles of generators and discriminators.
- Advanced GANs
- Exploring variations such as CGANs and CycleGANs for creative and practical applications.
- Understanding GANs
- Image Segmentation Techniques
- Semantic and Instance Segmentation
- Techniques for precise pixel-level image understanding using models like U-Net and Mask R-CNN.
- Segmentation Projects
- Application of segmentation techniques to real-world scenarios such as medical imaging or autonomous driving.
- Semantic and Instance Segmentation
- Capstone Project
- Project Design and Implementation
- Students will identify a problem statement, select appropriate datasets, and propose a solution using techniques learned throughout the course.
- Project Presentation and Evaluation
- Detailed presentation of their projects, including their approach, challenges faced, solutions implemented, and results achieved.
- Project Design and Implementation
- Course Review and Advanced Topics
- Review Sessions
- Recap of key concepts and technologies covered throughout the course.
- Emerging Trends in AI and Computer Vision
- Discussions on the latest research and potential future directions in deep learning and computer vision.
- Review Sessions
- Deep Dive into Text Processing and Linguistic Feature Extraction
- Advanced Text Manipulation
- Expanding on text preprocessing techniques using spaCy and NLTK.
- Advanced operations including custom tokenizers, stemmers, and lemmatizers.
- Semantic Analysis and Feature Engineering
- Extracting and utilizing linguistic features such as POS tags, dependency tags, and named entities for deeper semantic analysis.
- Practical exercises on feature engineering for NLP.
- Advanced Text Manipulation
- Vector Representations and Embeddings
- Word Embeddings and Document Vectors
- In-depth exploration of word embeddings with word2vec and GloVe. Application of these techniques for generating document-level embeddings.
- Implementing and comparing different embedding models using Gensim.
- Contextual Embeddings and Transformers
- Understanding the shift from static to contextual embeddings.
- Deep dive into the mechanics of transformers and their superiority in capturing contextual relationships.
- Word Embeddings and Document Vectors
- Mastering Transformers for NLP
- Implementation of Transformer Models
- Hands-on training with BERT, GPT-2, and GPT-3, focusing on model architecture and underlying technologies.
- Fine-tuning pre-trained models on specific NLP tasks such as sentiment analysis, text classification, and more.
- Advanced Applications of Transformers
- Applying transformers to tasks like neural machine translation, summarization, and question answering.
- Introduction to multilingual transformers and their applications.
- Implementation of Transformer Models
- Specialized NLP Tasks
- Text Classification and Sentiment Analysis
- Building sophisticated models for text classification including multi-label and hierarchical classification systems.
- Sentiment analysis models that can detect nuances and context in text.
- Named Entity Recognition (NER) and POS Tagging*
- Advanced techniques in NER and POS tagging using state-of-the-art transformer models.
- Customizing NER models to recognize specialized entities specific to domains like healthcare or finance.
- Text Classification and Sentiment Analysis
- Generating Human-like Text and Creative NLP Applications
- Generative Text Models
- Exploring the capabilities of generative models like GPT-3 for creating believable human-like text.
- Implementing models for tasks such as content generation, dialogue systems, and creative writing.
- Zero-shot Learning and Transfer Learning in NLP
- Techniques for applying zero-shot learning and transfer learning paradigms to NLP tasks.
- Case studies on leveraging minimal data for effective NLP modeling.
- Generative Text Models
- Capstone Project
- Real-World NLP Solutions
- Students will identify a pressing NLP problem, develop a solution using advanced models, and integrate it into a realistic application.
- Projects might include developing a comprehensive legal document analysis system, building a cross-language information retrieval system, or creating an advanced sentiment analysis platform for monitoring brand health.
- Project Presentation and Peer Review
- Presentation of capstone projects to showcase innovative solutions to complex NLP challenges.
- Peer review sessions to critique methodologies and discuss alternative approaches.
- Real-World NLP Solutions
- Supplemental Learning
- Ongoing: Cutting-Edge NLP Research and Trends
- Regular updates on the latest NLP research, including seminars and webinars hosted by experts in the field.
- Discussions on ethical considerations and future directions in NLP technologies.
- Ongoing: Cutting-Edge NLP Research and Trends
- Introduction to Flask
- Overview of Flask
- What is Flask? Understanding its microframework structure.
- Setting up a Flask environment: Installation and basic configuration.
- First Flask Application
- Creating a simple app: Routing and view functions.
- Templating with Jinja2: Basic templates to render data.
- Overview of Flask
- Flask Routing and Forms
- Advanced Routing
- Dynamic routing and URL building.
- Handling different HTTP methods: GET and POST requests.
- Working with Forms
- Flask-WTF for form handling: Validations and rendering forms.
- CSRF protection in Flask applications.
- Advanced Routing
- Flask and Data Handling
- Integrating Flask with SQL Databases
- Using Flask-SQLAlchemy: Basic ORM concepts, creating models, and querying data.
- API Development with Flask
- Creating RESTful APIs to interact with machine learning models.
- Using Flask-RESTful extension for resource-based routes.
- Integrating Flask with SQL Databases
- Introduction to FastAPI
- Why FastAPI?
- Advantages of FastAPI over other Python web frameworks, especially for async features.
- Setting up a FastAPI project: Installation and first application.
- FastAPI Routing and Models
- Path operations: GET, POST, DELETE, and PUT.
- Request body and path parameters: Using Pydantic models for data validation.
- Why FastAPI?
- Building APIs with FastAPI
- API Operations
- Advanced model validation techniques and serialization.
- Dependency injection: Using FastAPI’s dependency injection system for better code organization.
- Asynchronous Features
- Understanding async and await keywords.
- Asynchronous SQL database interactions using databases like SQLAlchemy async.
- API Operations
- Serving Machine Learning Models
- Integrating ML Models
- Building endpoints to serve predictions from pre-trained machine learning models.
- Handling asynchronous tasks within FastAPI to manage long-running ML predictions.
- Security and Production
- Adding authentication and authorization layers to secure APIs.
- Tips for deploying Flask and FastAPI applications to production environments.
- Integrating ML Models
- Introduction to Docker
- Overview of Docker
- Understanding what Docker is and the core concepts behind containers.
- Differences between Docker and traditional virtualization.
- Setting up Docker
- Installing Docker on various operating systems (Windows, macOS, Linux).
- Navigating Docker interfaces (Docker Desktop, Docker CLI).
- Overview of Docker
- Docker Basics
- Docker Images and Containers
- Understanding images vs. containers.
- Managing Docker images—pulling from Docker Hub, exploring Dockerfile basics.
- Running Containers
- Starting, stopping, and managing containers.
- Exposing ports, mounting volumes, and linking containers.
- Docker Images and Containers
- Docker Compose and Container Orchestration
- Introduction to Docker Compose
- Benefits of using Docker Compose.
- Writing a docker-compose.yml file for multi-container applications.
- Basic Orchestration
- Understanding the need for orchestration.
- Overview of Docker Swarm mode for managing a cluster of Docker Engines.
- Introduction to Docker Compose
- Docker for Data Science
- Creating a Data Science Work Environment
- Building a custom Docker image for data science environments.
- Including tools like Jupyter Notebook, RStudio, and popular data science libraries (Pandas, NumPy, Scikit-learn).
- Data Persistence in Containers
- Strategies for managing data in Docker, focusing on non-volatile data storage.
- Creating a Data Science Work Environment
- Advanced Docker Applications in Data Science
- Deploying Machine Learning Models
- Containerizing machine learning models for consistent deployment.
- Using Docker containers to deploy a model to a production environment.
- Best Practices and Security
- Understanding Docker security best practices.
- Maintaining and updating data science Docker environments.
- Deploying Machine Learning Models
- Capstone Project
- Project Implementation
- Apply the skills learned to containerize a data science project. This could involve setting up a full data processing pipeline, complete with a web interface for interacting with a machine learning model.
- Documentation and Presentation
- Document the Docker setup process and challenges encountered.
- Present the project, highlighting the benefits of using Docker in data science workflows.
- Project Implementation
- Evaluation
- Practical Tests
- Hands-on tasks to reinforce weekly topics, ensuring practical understanding and capability.
- Final Assessment
- A comprehensive test covering all topics from image creation to deployment and orchestration, assessing both theoretical knowledge and practical skills.
- Practical Tests
- Introduction to Redis
- Overview of Redis
- What is Redis and why is it used? Understanding its role as an in-memory data structure store.
- Key features of Redis: speed, data types, persistence options, and use cases.
- Installation and Setup
- Quick guide on installing Redis on different operating systems (Windows, Linux, macOS).
- Starting the Redis server and basic commands through the Redis CLI.
- Overview of Redis
- Redis Data Types and Basic Commands
- Key-Value Data Model
- Introduction to Redis’ simple key-value pairs; commands like SET, GET, DEL.
- Advanced Data Types
- Lists, Sets, Sorted Sets, Hashes, and their associated operations.
- Practical examples to demonstrate each type: e.g., creating a list, adding/removing elements, accessing elements.
- Key-Value Data Model
- Redis in Application
- Caching Concepts
- Explaining caching and its importance in modern applications.
- How Redis serves as a cache: advantages over other caching solutions.
- Implementing Basic Caching
- Setting up a simple cache: handling cache hits and misses.
- Expiration and eviction policies: how to manage stale data in Redis.
- Caching Concepts
- Introduction to Kafka and Confluent Platform
- Overview of Kafka, introduction to Confluent Kafka, and its ecosystem.
- Hands-on setup of Confluent Kafka, including creating your first Kafka producer and consumer.
- Advanced Kafka Producers and Consumers
- Deep dive into Kafka producers, including serialization and partitioning.
- In-depth exploration of Kafka consumers, understanding consumer groups, and offsets.
- Data Integration with Kafka Connect and Schemas
- Setup and use Kafka Connect for seamless data import/export.
- Introduction to Schema Registry and implementing Avro schemas.
- Real-Time Data Processing with Kafka Streams and ksqlDB
- Building streaming applications using Kafka Streams.
- Leveraging ksqlDB for real-time data analysis.
- Data Engineering for Deep Learning
- Overview of essential data engineering tools and their roles in handling large-scale data.
- Integration of Kafka with deep learning models and real-time analytics.
- Capstone Project
- Students will implement a project integrating Kafka with a real-time deep learning model, exploring scenarios such as image or speech recognition using live data streams.
- The course concludes with a review of the projects, feedback sessions, and discussions on potential improvements and real-world applications of the skills learned.
- Learning Outcomes
- Upon completion, participants will:
- Understand the architecture and components of Kafka and the Confluent platform.
- Be proficient in setting up and managing Kafka producers, consumers, and streams.
- Have practical experience with Kafka’s integration into data pipelines and real-time data processing systems.
- Be able to assess and implement Kafka solutions in data science applications, particularly in enhancing the capabilities of deep learning models with real-time data feeds.
- Upon completion, participants will:
Transform Your Skills: Enroll Now to Learn
Speak to our career counsellor for detailed overview of SCAI Data Science Course. For a more detailed explanation of what SCAI Data Science can offer don’t hesitate to contact our team today. We will set up a personalized demo and meeting with our experts and counsellors who can answers questions you may have Let’s take your career to next level with SCAI!! If you’re interested in upskilling your career with a data science course, SCAI offers a comprehensive program designed to equip you with the necessary skills knowledge for success in this field.