Python Data Structures and Algorithms Mastery
Welcome to our comprehensive course on Data Structures and Algorithms using Python, designed for learners from various backgrounds aiming to strengthen their problem-solving and programming skills. This course is ideal for both beginners and those who have some programming experience but want to delve deeper into the intricacies of data structures and algorithms.
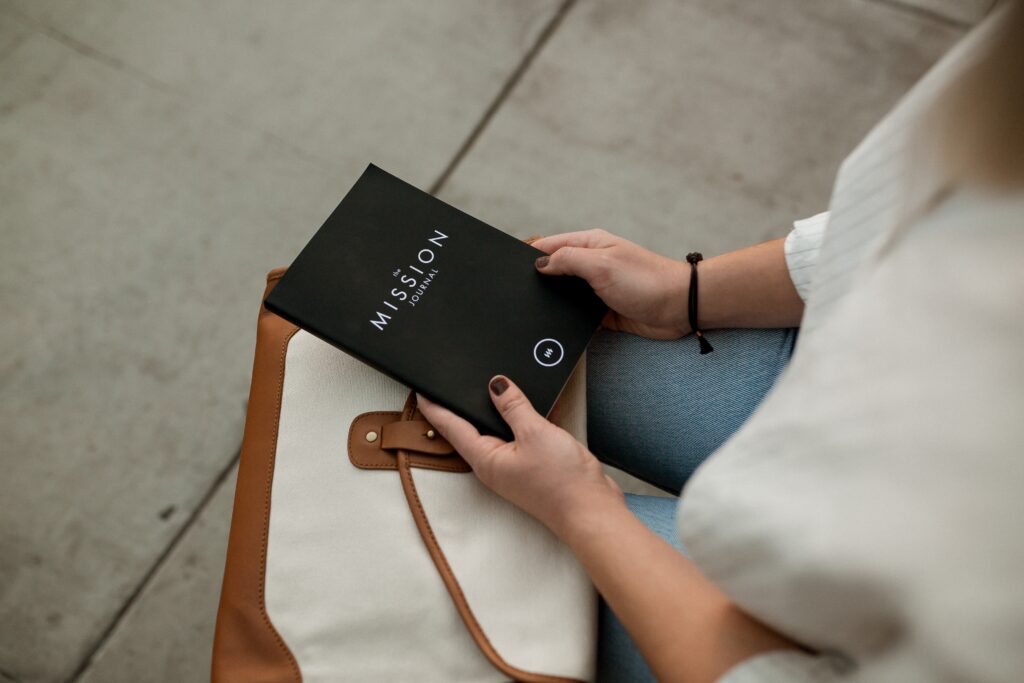
Skills You Will Gain
Proficiency in Python Programming
Understanding of Basic to Advanced Data Structures
Algorithm Design and Analysis
Problem-solving and Critical Thinking Skills
Implementation of Algorithms in Python
Optimization Techniques for Better Performance
Preparing for Coding Interviews and Competitions
This course includes
- 1 : 1 Session
- 100% Placement Assistance
- 16 Weeks
- Real TIme Project Training
Syllabus Overview
Data Structures and Algorithms in Python
This course focuses on building a strong foundation in data structures and algorithms, crucial for any aspiring software developer or programmer.
- Refreshing Python Fundamentals
- Arrays and Lists in Python
- Stack and Queue Implementation
- Linked Lists: Single, Double, and Circular
- Basic Sorting Algorithms: Bubble Sort, Insertion Sort, Selection Sort
- Trees: Binary Trees, Binary Search Trees, AVL Trees, and Heap
- Graphs: Representations and Basic Graph Algorithms
- Hash Tables and Hashing Functions
- Advanced Sorting Algorithms: Merge Sort, Quick Sort, Heap Sort
- Searching Algorithms: Binary Search, Depth-First Search, Breadth-First Search
- Time and Space Complexity Analysis
- Divide and Conquer Algorithms
- Dynamic Programming and Greedy Algorithms
- Backtracking Techniques
- Graph Algorithms: Dijkstra’s, A* Search, Kruskal’s, Prim’s
- Applying Data Structures and Algorithms to Solve Real-World Problems
- Algorithm Design Patterns and Techniques
- Preparing for Technical Interviews
- Competitive Programming Strategies
- Best Practices in Python Programming
Transform Your Skills: Enroll Now for Master Python
Throughout the course, participants will engage in hands-on coding exercises, challenging problem sets, and real-world projects. These practical experiences are designed to reinforce learning and develop the skills needed to apply data structures and algorithms effectively in various scenarios. By the end of the course, you will have gained a deep understanding of data structures and algorithms in Python, preparing you for advanced programming challenges, technical interviews, and competitive programming contests.